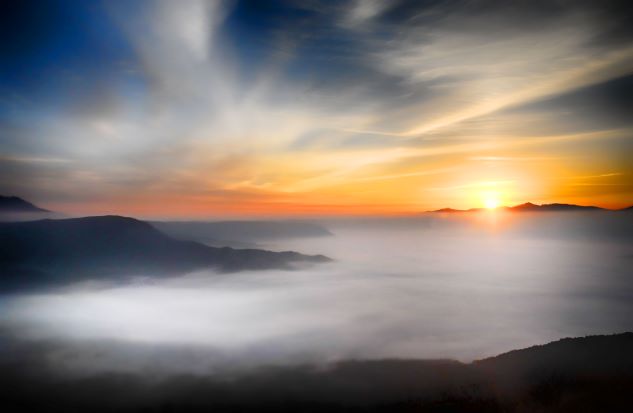
In this tutorial, I will show you how to use Appdaemon to create a custom sensor in Home Assistant that displays the day of the week. You may be interested in creating such a custom sensor if you have the need to show the day on a smart display, or simply as a utility in your automation toolbox to incorporate as a trigger into your other automations.
We will need to first import two necessary modules to code the automation: appdaemon.plugins.hass.hassapi and datetime.
import appdaemon.plugins.hass.hassapi as hass
import datetime
Next, we create a class called DayMonitor that inherits from hass.Hass. This class will contain the code for our sensor.
class DayMonitor(hass.Hass):
Inside the class, we define the Appdaemon initialize method. This method is called automatically when the app is loaded.
We are tracking when the sun rises as a trigger to ascertain when the day changes. Thus, inside the initialize method, we set the sun variable to the entity ID of our sun sensor. We also use the listen_state method to register a callback that will be triggered when the state of the sun sensor changes to “above_horizon”, which indicates that the sun has risen. This callback is defined in the below day_change_monitor_sun_rise method.
def initialize(self):
self.sun = "sun.sun"
self.listen_state(self.day_change_monitor_sun_rise, self.sun, new="above_horizon")
Next, inside the day_change_monitor_sun_rise method, we get the current day of the week using the code datetime.datetime.today().weekday(). We then use an if-else block to set the state of our “sensor.today” sensor to the corresponding day of the week. We also use the log method to log the current day and the new state of the sun sensor, though this last step is optional.
def day_change_monitor_sun_rise(self, entity, attribute, old, new, kwargs):
self.day = datetime.datetime.today().weekday()
if self.day == 0:
self.set_state("sensor.today", state = "Monday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 1:
self.set_state("sensor.today", state = "Tuesday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 2:
self.set_state("sensor.today", state = "Wednesday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 3:
self.set_state("sensor.today", state = "Thursday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 4:
self.set_state("sensor.today", state = "Friday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 5:
self.set_state("sensor.today", state = "Saturday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
This is all of the code. Again, you can use this custom sensor in other automations to create smarter home automations with conditional statements based on the current day.
Below is the Complete Uncommented Code
import appdaemon.plugins.hass.hassapi as hass
import datetime
class DayMonitor(hass.Hass):
def initialize(self):
self.sun = "sun.sun"
self.listen_state(self.day_change_monitor_sun_rise, self.sun, new="above_horizon")
def day_change_monitor_sun_rise(self, entity, attribute, old, new, kwargs):
self.day = datetime.datetime.today().weekday()
self.log(self.day)
self.log(new)
if self.day == 0:
self.set_state("sensor.today", state = "Monday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 1:
self.set_state("sensor.today", state = "Tuesday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 2:
self.set_state("sensor.today", state = "Wednesday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 3:
self.set_state("sensor.today", state = "Thursday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 4:
self.set_state("sensor.today", state = "Friday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 5:
self.set_state("sensor.today", state = "Saturday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))
elif self.day == 6:
self.set_state("sensor.today", state = "Sunday")
self.log("Today is "+ self.get_state("sensor.today", attribute = "state"))