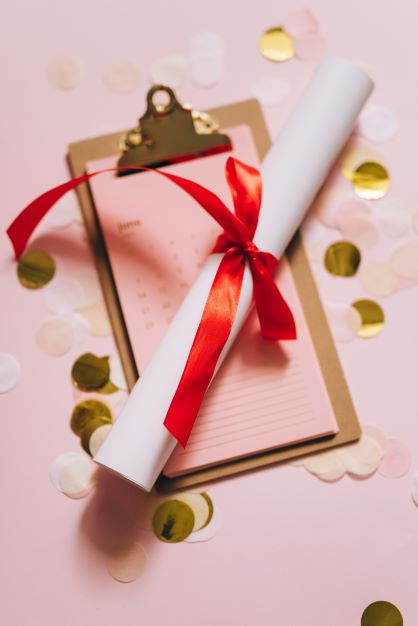
Have you ever found yourself configuring an automation in Home Assistant and you needed to find out what day is today, yesterday, and tomorrow, or whether any of those days fell on a U.S. holiday? That kind of information is unfortunately not intuitively available in Home Assistant without additional programming; yet having access to such information is crucial for creating dynamic automations in Home Assistant. In this article, I will show you how to use Appdaemon to access that information to program smarter home automations with more complex logics.
Part I
If you have not yet done so, you will first need to install and configure Appdaemon in Home Assistant. It may also be helpful to familiarize yourself with the Appdaemon API documentation if you are new to configuring smart home automations with the tool.
Part II
We will be using an external Python library in our automation. So, before we do anything else, let’s go ahead and import that library into Appdaemon. If you do not import the external library, your code will not run.
The Python library we need to import into Appdaemon is called Pandas. We will be using Pandas to retrieve the calendar consisting of all U.S. federal holidays.
The procedure for importing a library into Appdaemon is as follows:
- Go to “settings” in Home Assistant
- Click on “Add-ons Run extra applications next to Home Assistant”
- Click on “Appdaemon.” If you have configured the integration correctly, you should see Appdaemon in the list of running applications in your Home Assistant instance.
- Once you have the Appdaemon screen loaded, click on “Configuration.”
- In the box labeled “Python Packages.,” type-in “pandas,” which is the name of the library we want to import.
- Then, click “save.”
- At this time, Appdaemon should restart to allow the library to be installed. If it does not restart on its own, you will need to restart the integration manually by clicking on the “information Tab and then click on “Restart.”
- Appdaemon will take a few minutes to install the library upon restarting. You can click on the “log” tab to monitor the progress of the install.
Part III
Now that you have configured the Appdaemon integration and installed the associated pandas library, you are ready to start coding. I am going to walk you through the code line by line. The “#” sign is Python’s code to indicate that the line has been commented. At the end of the article, I will place an uncommented version of the automation for your use.
Please note: After creating the first method, the explanation gets a bit repetitive because at that point the code is mostly similar to the prior code.
You are free to use a Notepad document or an actual programming environment such as Visual Studio to write your code. To set up an Appdaemon App, at minimum you will need to do three things:
- Import the Appdaemon library
- Create a subclass of the library, and
- Initialize the app.
I covered the procedure for configuring and registering an Appdaemon app in this YouTube video. If after viewing the video you still need additional assistance, please consult the Appdaemon API documentation, or send me a message and I will try to help.
# Importing the Appdaemon library
import appdaemon.plugins.hass.hassapi as hass
# Importing the Python date module
import datetime
from datetime import date, timedelta
# Importing the Python calendar module
import calendar
# Importing the U.S. federal holidays calendar from the Pandas library
from pandas.tseries.holiday import USFederalHolidayCalendar
#Creating the class for my automation. I gave it the name “DateUtility.”
class DateUtility(hass.Hass):
# Initializing my app.
def initialize(self):
Part IV
You have by now created and initialized the Appdaemon app. Next, we will be writing the logic for our automation, which is to find out what day is today, what day was yesterday, and what day will be tomorrow, and whether any of those days fall on a U.S. holiday. I am going to store each date in a variable in my initialize method, as follows:
# Saving today’s date in a class variable so I can use it in my methods later on.
self.current_date = date.today()
# Saving yesterday’s date in a class variable so I can use it in my methods later on. Please note how I am using today’s date to retrieve yesterday’s date.
self.yesterday = self.current_date -datetime.timedelta(days=1)
# Saving tomorrow’s date in a class variable so I can use it in my methods later on. Please note how I am using today’s date to retrieve yesterday’s date.
self.tomorrow = self.current_date + datetime.timedelta(days=1)
Part V
We have been able to retrieve the actual dates for today, yesterday, and tomorrow. However, that is not enough. We need to code several methods using the dates to also retrieve each day by their respective name, such as Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, or Sunday. We will start by getting the day by name for today’s date.
#Today
def day_today(self):
# We are using the calendar module to get the day by name and saving it in a variable called “today_day_name.”
today_day_name = calendar.day_name[self.current_date.weekday()]
# I am adding a message to the Appdaemon log for testing purposes
self.log(today_day_name)
# Getting the method to return the day by name
return today_day_name
# yesterday
def day_yesterday(self):
# We are using the calendar module to get yesterday by name and saving it in a variable called “yesterday_day_name.”
yesterday_day_name = calendar.day_name[self.yesterday.weekday()]
# I am adding a message to the Appdaemon log for testing purposes
self.log(yesterdayday_day_name)
# Getting the method to return yesterday by name
return yesterdayday_day_name
#Tomorrow
def day_tomorrow(self):
# We are using the calendar module to get yesterday by name and saving it in a variable called “tomorrow_day_name.”
tomorrow_day_name = calendar.day_name[self.tomorrow.weekday()]
# I am adding a message to the Appdaemon log for testing purposes
self.log(tomorrow_day_name)
# Getting the method to return tomorrow by name
return tomorrow_day_name
Part VI
This is progress. We now have written the logic to get today, yesterday, and tomorrow’s dates by their respective names. One of the information that is often useful, such as in configuring a trash-day pickup announcement automation, is knowing whether any of those days fell on a holiday, which in some places would result in the pick up date being pushed to the next day. Let’s code a method to tell us that information for each of the day. Why not?
#Today
def is_today_holiday(self):
# Using the Pandas library to access the US list of holidays an saving it in a variable call “cal.”
cal = USFederalHolidayCalendar()
# Defining the year for which I need to know the holidays
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
# Using a “for loo” to loop over each one of the holidays in the holiday object and compare it to the date I’m interested in knowing if it falls on a holiday.
for holiday in holidays:
# Checking for Today’s date
if holiday.date() == self.current_date:
# If today is a holiday, it will return True
return True
else:
# If today is not a holiday, it will return False
return False
#Yesterday
def is_yesterday_holiday(self):
# Using the Pandas library to access the US list of holidays an saving the object in a variable call “cal.”
cal = USFederalHolidayCalendar()
# Defining the year for which I need to know the holidays
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
# Using a “for loo” to loop over each one of the holidays in the holiday object and compare it to the date I’m interested in knowing if it falls on a holiday.
for holiday in holidays:
# Checking for yesterday’s date
if holiday.date() == self.yesterday:
# If yesterday was a holiday, it will return True
return True
else:
# If yesterday was not a holiday, it will return False
return False
#tomorrow
def is_tomorrow_holiday(self):
# Using the Pandas library to access the US list of holidays an saving the object in a variable call “cal.”
cal = USFederalHolidayCalendar()
# Defining the year for which I need to know the holidays
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
# Using a “for loo” to loop over each one of the holidays in the holiday object and compare it to the date I’m interested in knowing if it falls on a holiday.
for holiday in holidays:
# Checking for tomorrow’s date
if holiday.date() == self.tomorrow:
# If tomorrow will fall on a holiday, it will return True
return True
else:
# If tomorrow will not fall on a holiday, it will return False
return False
Part VII
Below is the complete code without any comments:
import appdaemon.plugins.hass.hassapi as hass
import datetime
from datetime import date, timedelta
import calendar
from pandas.tseries.holiday import USFederalHolidayCalendar
class DateUtility(hass.Hass):
def initialize(self):
self.current_date = date.today()
self.yesterday = self.current_date -datetime.timedelta(days=1)
self.tomorrow = self.current_date + datetime.timedelta(days=1)
# Today
def day_today(self):
today_day_name = calendar.day_name[self.current_date.weekday()]
self.log(today_day_name)
return today_day_name
# Yesterday
def day_yesterday(self):
yesterdayday_day_name = calendar.day_name[self.yesterday.weekday()]
self.log(yesterdayday_day_name)
return yesterdayday_day_name
#Tomorrow
def day_tomorrow(self):
tomorrow_day_name = calendar.day_name[self.tomorrow.weekday()]
self.log(tomorrow_day_name)
return tomorrow_day_name
#Today holiday
def is_today_holiday(self):
cal = USFederalHolidayCalendar()
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
for holiday in holidays:
if holiday.date() == self.current_date:
return True
else:
return False
# yesterday holliday
def is_yesterday_holiday(self):
cal = USFederalHolidayCalendar()
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
for holiday in holidays:
if holiday.date() == self.yesterday:
return True
else:
return False
#tomorrow holiday
def is_tomorrow_holiday(self):
cal = USFederalHolidayCalendar()
holidays = cal.holidays(start="2022-01-01", end="2022-12-31")
for holiday in holidays:
if holiday.date() == self.tomorrow:
return True
else:
return False
Part VIII
To test each method, you can log a message by calling the method in the Appdaemon log as follows:
self.log(self.day_today())
The “self.day_today()” is one of the methods we have just written. The self.log() method is an Appdaemon method that is equivalent to the print method in Python.
For testing purposes, you can place the “self.log(self.day_today())” in the initialize method so that the code runs every time you save the file. It will return the name of the particular day: today, yesterday, or tomorrow, or if you are calling the holiday methods, it will return True or False for each of the methods.
Then, open your Appdaemon log and observe whether the method fired successfully after saving the file containing your code.
The procedure for opening your Appdaemon log is similar to the procedure that you initially followed to install and configure Appdaemon.
- Go to “Settings” in Home Assistant.
- Click on “Add-ons Run extra applications next to Home Assistant.”
- Click on “Appdaemon.”
- Once you have the Appdaemon screen loaded, click on the “log” tab.
Please consider subscribing to our newsletter so you are able to receive new smart home automations such as this one in your inbox.